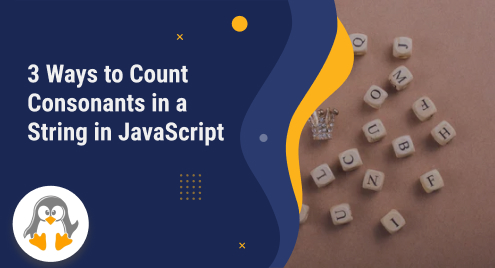
3 Ways to Count Consonants in a String in JavaScript
While working with strings in JavaScript, it’s common to come into scenarios where developers need to analyze the contents of a string and extract certain information. One such example is counting the consonants in a specified string. Unlike vowels, which are generated with relatively unrestricted airflow, consonants involve some type of restriction or constriction.
Consonants in the English language include letters like ‘b’, ‘c’, ‘d’, ‘f’, and so on. By counting the consonants in a string, developers can obtain insight into the phonetic composition of the text or do text analysis tasks.
This article will illustrate the methods for counting consonants in a string in JavaScript.
How to Count Consonants in a Specified String in JavaScript?
To count consonants in a string in JavaScript, use the following JavaScript approaches:
- for loop
- Regular expression
- split() method
Method 1: Count Consonants in a String Using “for” Loop
For counting consonants in a string, use the “for” loop. It is an iterative method or a control flow statement in JavaScript that allows the execution of a block of code repeatedly based on a specified condition.
Syntax
The following syntax is used for using the “for” loop:
for (initialization; condition; iteration) { // code to be executed } |
---|
Example
In the given example, first, we will define a function named “consonantsInString” for counting consonants in a string. It takes a string as an argument:
function consonantsInString(str) { var count = 0; for (var i = 0; i < str.length; i++) { if (str[i] !== "a" && str[i] !== "e" && str[i] !== "i" && str[i] !== "o" && str[i] !== "u" && str[i] !== " ") { count++; } } return (count); } |
---|
In the above-defined function:
- First, declare a variable “count” and initialize it with the value “0”.
- Then, iterate the string until its length using the “for” loop where to check every string character and increment the count if it is not a vowel (‘a’, ‘e’, ‘i’, ‘o’, ‘u’).
Now, call the function in the “console.log()” method by passing a string as a parameter:
console.log(consonantsInString("JavaScript is used for developing web pages")); |
---|
The output displays “23” which is the count of the consonants in a specified string:
Method 2: Count Consonants in a String Using “Regular Expression”
The commonly used way to count the consonants in a string is “Regular expressions”. Use the “match()” method with the specified pattern to match the regex pattern with the string.
Syntax
The given syntax is used for the match() method with a regular expression:
match(pattern); |
---|
Example
In the given example, we will take a string as an input in a function and define a regular expression that matches all consonant characters. In the regex pattern, the “gi” flag makes the global match. Then, call the “match()” method on the input string with the regular expression to find all the consonant matches. If there are any matches, the function outputs the number of matches; otherwise, it gives 0:
function consonantsInString(str) { const consonantsRegex = /[bcdfghjklmnpqrstvwxyz]/gi; const checkString = str.match(consonantsRegex); const count = checkString ? checkString.length : 0; return count; } |
---|
Call the function by passing the string as a parameter:
consonantsInString("JavaScript is used for developing web pages"); |
---|
Output
Method 3: Count Consonants in a String Using “split()” Method
You can also use the “split()” method for counting consonants in a string. It is not typically used to count consonants in a string, it can be leveraged in combination with other techniques to achieve the desired result.
Here, we will use the “toLowerCase()” method with the “split()” method. It will ensure that both uppercase and lowercase consonants are counted consistently and split the string into an array of individual characters.
Example
Here, we will define a function that accepts a string as an input parameter. Then, create a string of consonants that will match the array of individual characters of the string. If the string elements include in the array of characters, it will increment the count:
function consonantsInString(string) { const consonants = ("bcdfghjklmnpqrstvwxyz"); const lowercaseString = string.toLowerCase(); const stringCharacters = lowercaseString.split(''); let count = 0; stringCharacters.forEach(character => { if (consonants.includes(character)) { count++; } }); return count; } |
---|
Declare a variable “string” that stores a string:
const string = "JavaScript is used for developing web pages"; |
---|
Pass the string in the function “consonantsInString()” and store the resultant count in the variable “consonantCount”:
const consonantCount = consonantsInString(string); |
---|
Finally, print the count on the console using the “console.log()” method:
console.log(consonantCount); |
---|
Output
You can also specify the consonants in an array:
const consonants = ['b', 'c', 'd', 'f', 'g', 'h', 'j', 'k', 'l', 'm', 'n', 'p', 'q', 'r', 's', 't', 'v', 'w', 'x', 'y', 'z']; |
---|
Output
That’s all about counting the consonants in a string in JavaScript.
Conclusion
To count consonants in a string in JavaScript, use the “for” loop, “Regular expression”, or the “split()” method with the “toLowerCase()” and “include()” methods. “Regular expression” is the commonly used method for counting the consonants in a string in JavaScript. This article illustrated the methods for counting consonants in a string in JavaScript.