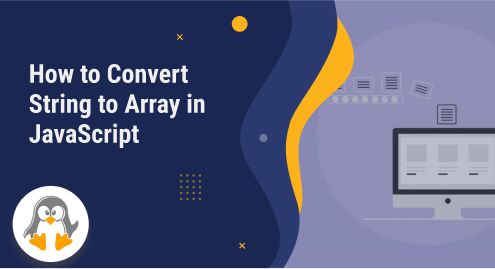
How to Convert String to Array in JavaScript
While working with JavaScript, the conversion of string to array is a common task. It is useful while manipulating individual characters or substrings within a string. There are several predefined approaches for the string-to-array conversion in JavaScript including the “split()” method, the “from()” method of an Array object, and so on.
This tutorial will describe the methods of string-to-array conversion in JavaScript.
How to Convert/Transform a String to an Array Using JavaScript?
The following approaches are utilized for the conversion of a string into an array:
- split() method
- Array.from() method
- Object.assign() method
- Spread operator
Method 1: Using the “split()” Method For Converting/Transforming String to Array
For the conversion of a string to an array in JavaScript, use the “split()” method. It splits the defined string into a substring array based on a particular separator. If you haven’t added any separator, the split() method splits/divides the string into an array of individual characters.
Syntax
The given syntax is used for the split() method without using any separator:
string.split(""); |
---|
For using a separator, pass the particular value or character to the method such as “,”, “:”, and so on.
Example
Here, in the given example, we will split the string into individual characters without using any separator. First, we will create a string:
const string = "JavaScript"; |
---|
Call the split() method on the string to convert it into an array of characters:
const array = string.split(""); |
---|
Print the resultant array on the console:
console.log(array); |
---|
The output indicates that the split() method has successfully converted the string into an array:
Method 2: Using “Array.from()” Method For Converting/Transforming String to Array
You can also utilize the “Array.from()” method for string-to-array conversion. It is a static method in JavaScript that converts an iterable object or an object that looks like an array into a new instance of an array. It takes/accepts a string as an argument for converting it into an array.
Syntax
The following syntax is utilized for the Array.from() method:
Array.from(string) |
---|
Example
Call the Array.from() method by passing a string as an argument and printing the resultant array on the console using the “console.log()” method:
const array = Array.from(string); console.log(array); |
---|
Output
Method 3: Using “Object.assign()” Method For Converting/Transforming String to Array
Use the “Object.assign()” method. Like Array.from(), this method is also a static method that copies the values of all enumerable properties from one or more source objects to a target object.
Syntax
The provided syntax is used for the Object.assign() method:
Object.assign(target, source); |
---|
Example
Call the Object.assign() method and pass the array as a target and string as a source. It will convert the source into a target object:
const array = Object.assign([], string); console.log(array); |
---|
Output
Method 4: Using “Spread Operator” For Converting/Transforming String to Array
Another way for converting a string to an array is to use the spread operator (…). It is used to expand a string into individual elements, which can then create a new array.
Syntax
Use the following syntax for the conversion of a string to an array with the help of the spread operator:
[...string] |
---|
Example
Pass the string with the spread operator in an array to expand a string into individual characters, and then, print it on the console:
const array = [...string]; console.log(array); |
---|
Output
We have compiled all the best solutions for converting string to an array in JavaScript.
Conclusion
For string-to-array conversion, JavaScript provides predefined methods including the “split()” method, “Array.from()” method, “Object.assign()” method or the “Spread operator”. These methods allow manipulation and access of substrings or individual characters in a string. This tutorial described the string-to-array conversion methods in JavaScript.