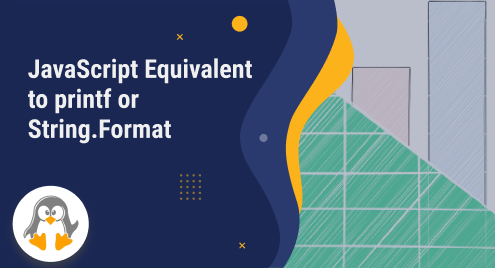
JavaScript Equivalent to Printf or String.Format
The “printf” and “String.Format” are commonly used in programming languages such as C, C++, C#, Java, Python, and so on. These functions or methods are used for formatting strings by specifying placeholders and dynamically substituting values. These functions offer a flexible and convenient way to control the output format of strings.
This tutorial will describe the equivalent of printf or String.Format in JavaScript.
What is printf() function?
The “printf” function originated in the C programming language and has been adopted by many other languages. It takes a format string containing placeholders usually marked with a “%” symbol and a corresponding list of values to substitute into those placeholders.
The format string includes format specifiers that define the desired formatting for each value, such as %d for integers, %f for floating-point numbers, %s for strings, and so on.
What is the String.Format() method?
“String.Format” is a method available in some languages such as C#, Java, and Python. It allows developers to format strings by using placeholders enclosed in curly braces ({}) and specifying the values to substitute in a separate argument list. While JavaScript does not have a pre-built equivalent to “printf” and “String.Format”, there are alternative methods and libraries that provide similar functionality.
What is the Equivalent of printf or String.Format in JavaScript?
JavaScript does not support “printf” or “String.Format” but it provides some alternatives to achieve similar functionality. As an equivalent of “printf” or “String.Format” in JavaScript use the following approaches:
- template literals
- regular expressions with String.prototype Object
Method 1: Use “template literals” as an Equivalent of printf or String.Format in JavaScript
JavaScript’s “template literals”, were introduced in ES6 (ECMAScript 2015). It provides a concise way for creating formatted strings. It allows embedding expressions and variables directly within the string by using placeholders, denoted by backticks (`). Placeholders are indicated by using the “${}” syntax.
Example
Create two variables “a” and “b” that stores strings “JavaScript” and “Website”:
var a = "JavaScript"; var b = "Website"; |
---|
Use the template literals to create a message string by incorporating the values of “a” and “b” within the placeholders as ${a} and ${b} respectively:
var formattedString = `I am interested to learn ${a} for creating a dynamic ${b}.`; |
---|
Print the message string on the console with the help of the “console.log()” method:
console.log(formattedString); |
---|
As you can see the output has been successfully formatted the string by embedding values of the variables:
Similarly, for displaying message strings on DOM instead of the console, use the “document.write()” method.
Method 2: Use “Regular Expressions” With “String.prototype” Object as Equivalent of printf or String.Format in JavaScript
Combine “regular expressions” with the “String.prototype.format” method to achieve a similar effect to “printf” or “String.Format” for string formatting. Formatted strings can be created by using placeholders in the string and regular expressions to match and replace those placeholders with dynamic values.
Example
First, extend the “String.prototype” object with a custom “format” method. It will take any number of arguments and utilize a loop for iterating over the arguments. It creates a regular expression dynamically with the placeholder index, such as \{0\} for the first argument, \{1\} for the second argument, and so on.
Then, use the “String.prototype.replace()” method for finding and replacing each placeholder in the original string with the corresponding argument value. The “g” flag in the regular expression ensures that all instances of the placeholder are replaced. Finally, return the formatted string:
String.prototype.format = function (...args) { let formattedString = this; for (let i = 0; i < args.length; i++) { const regex = new RegExp(`\\{${i}\\}`, 'g'); formattedString = formattedString.replace(regex, args[i]); } return formattedString; }; |
---|
Print the string with arguments by calling the function “format”:
console.log("I love to learn {0} for creating a {1} {2}.".format("JavaScript", "dynamic", "websites")); |
---|
Output
We have compiled all the relevant instructions for the equivalent of printf and String.format in JavaScript.
Conclusion
JavaScript does not support “printf” or “String.Format” but it provides some alternatives to achieve similar functionality by using the “template literals” or “regular expressions” with “String.prototype” Object. Template literal is a powerful way to format strings in JavaScript. It offers a convenient and adaptive alternative to printf or String.Format found in other programming languages. This tutorial described the equivalent of printf or String.Format in JavaScript.